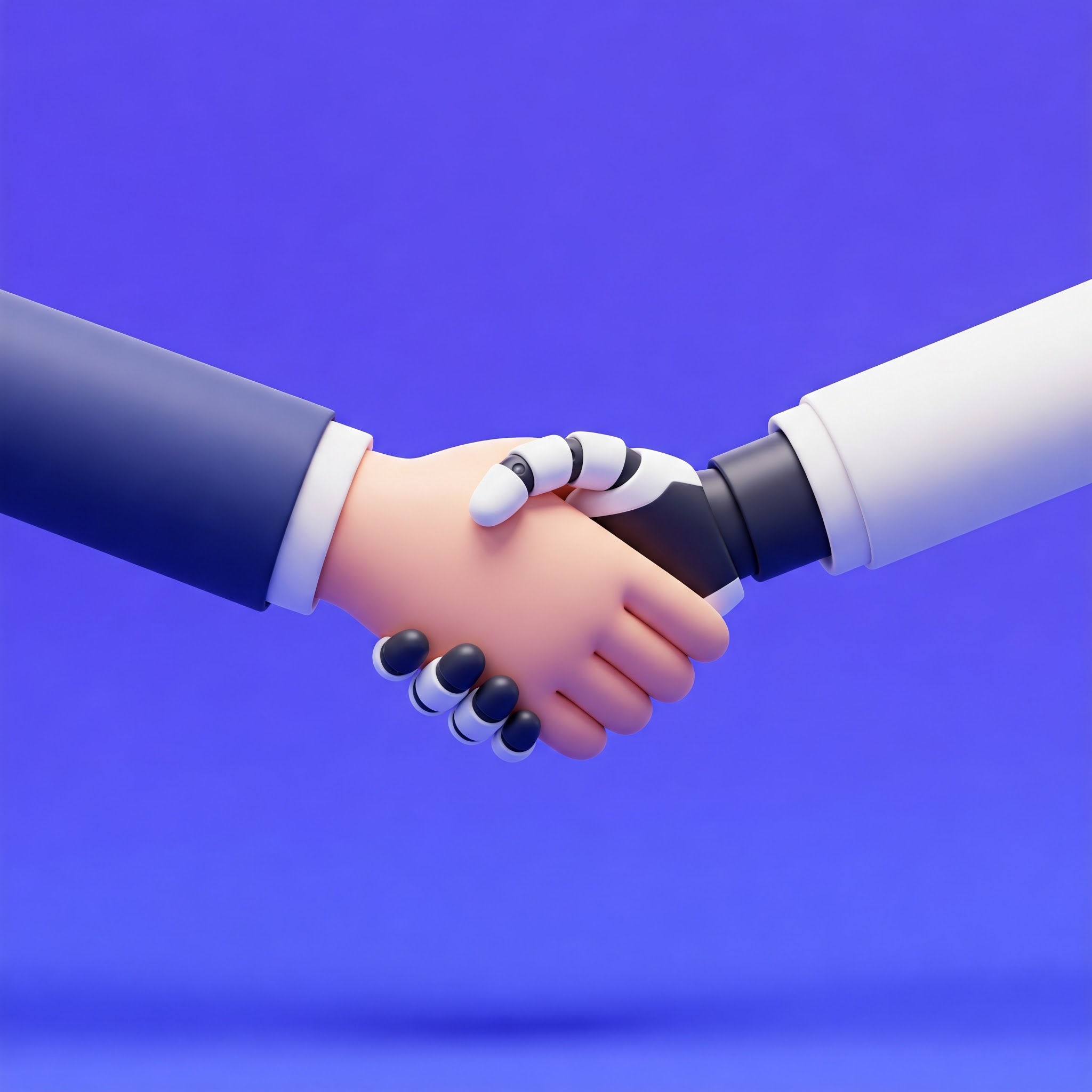
The Anti-Spaghetti Manifesto: A Ride through the Perils of Clean Code!
Discover the secrets of writing clean code that is easy to understand, maintain, and impress your fellow developers (not really).
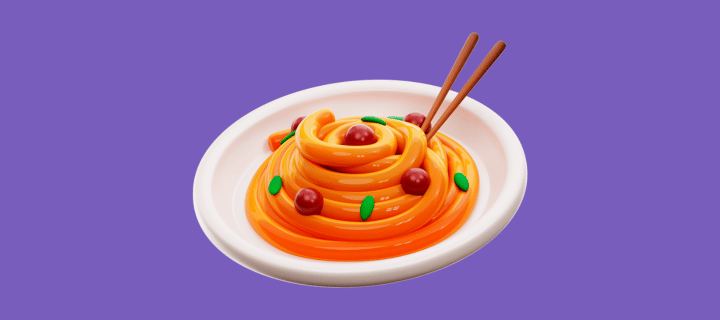
TL;DR:
- Code reviews foster collaboration, growth, and better code quality.
- Tools assist, but human judgment is essential in reviews.
- Professionalism extends beyond code reviews to all aspects of software development.
- Clean code principles include managing complexity, meaningful naming, and effective error handling.
- Unit tests should be focused, readable, and maintainable.
- Well-designed classes follow SOLID principles with high cohesion and low coupling.
- Concurrency demands proper synchronization, thread safety, and robust error handling.
- Clean design adheres to SOLID, Law of Demeter, and favors composition over inheritance.
Navigating clean code: from chaos to clarity
Imagine this: You’re staring at a screen filled with tangled, unstructured code—more like a plate of spaghetti than a well-thought-out solution. Every developer encounters this challenge at some point. Writing clean code isn’t just about aesthetics; it’s about maintainability, readability, and collaboration.
Welcome to The Anti-Spaghetti Manifesto, a practical guide to untangling complexity and writing code that’s easy to understand and improve.
The code review conundrum: balancing objectivity and empathy
Code reviews are essential to maintaining clean code. Done right, they foster learning and improve overall code quality. But reviews can also be daunting—harsh feedback can feel personal, even when it isn’t.
Instead of seeing code reviews as a critique of your abilities, treat them as a conversation. Engaging with reviewers, asking for clarification, and discussing alternative approaches not only improves the code but also strengthens team collaboration. This guide breaks down how to make reviews more effective and less adversarial.
How to give effective code review feedback
When reviewing code:
- Understand the context: Instead of immediately pointing out flaws, ask why the developer took a certain approach.
- Frame suggestions constructively: Instead of “This is wrong,” try “Have you considered this approach for better readability?”
- Encourage best practices: Share insights on maintainability, performance, and security.
Feedback should empower, not discourage. Effective reviews lead to cleaner, more robust code while fostering a culture of learning and respect.
Pair programming: more than just two people coding together
Some see pair programming as inefficient, but it’s actually a powerful tool for knowledge sharing and debugging. Working through a problem with a colleague leads to:
- Faster problem-solving: Two perspectives can often break through coding roadblocks.
- Knowledge transfer: Less experienced devs learn best practices from senior engineers in real time.
- Better code quality: Immediate feedback prevents minor issues from becoming major refactors later.
If your team hasn’t experimented with pair programming, consider giving it a shot on complex or high-impact tasks.
Pushing beyond comfort zones: new paradigms and tools
Sticking to familiar tools is comfortable, but software development evolves rapidly. Whether it’s exploring functional programming, learning a new framework, or integrating AI into workflows (like PullFlow AI), stepping outside your comfort zone enhances problem-solving skills and keeps your skills sharp.
What makes code “clean”?
Clean code is easy to read, maintain, and modify. It isn’t just about making code work—it’s about making it work well. Key principles include:
- Managing complexity: Break down problems into small, focused components.
- Meaningful naming: Variables, functions, and classes should clearly express intent.
- Readability over cleverness: The best code is the one that another developer can easily understand and improve.
For a deeper dive into structuring clean, maintainable code, this article explores actionable strategies.
Naming: the underestimated skill
Clear naming eliminates the need for excessive comments and makes code self-explanatory. Follow these practices:
- Use descriptive names: Instead of
temp
, name variables based on their purpose (currentUserId
). - Follow conventions: Maintain consistency with established style guides (e.g., camelCase in JavaScript, snake_case in Python).
- Avoid misleading names: Ensure names accurately reflect behavior.
Functions: small, focused, and predictable
A function should do one thing well. Follow these guidelines:
- Keep functions short: If a function is doing multiple tasks, break it down.
- Follow the Single Responsibility Principle (SRP): Each function should serve one clear purpose.
- Minimize parameters: Too many arguments make functions hard to use and test.
- Avoid side effects: Functions should not unexpectedly alter external states.
To comment or not to comment?
Clean code reduces reliance on comments by making code self-explanatory. But when necessary, comments should:
- Explain why, not what: Good code tells what it does; comments should explain why a decision was made.
- Be updated alongside code changes: Outdated comments are worse than no comments at all.
Formatting: why structure matters
Consistent formatting makes reading and reviewing code easier. Good practices include:
- Consistent indentation and spacing
- Limiting line length for readability
- Logical grouping of code blocks with whitespace
- Following language-specific style guides
Understanding data structures, objects, and the Law of Demeter
A key aspect of clean code is minimizing unnecessary dependencies. The Law of Demeter suggests that objects should only interact with their immediate dependencies, preventing excessive coupling and improving maintainability.
Handling errors effectively
Error handling should be explicit, meaningful, and separated from core logic. Best practices include:
- Using clear error messages
- Logging errors for debugging
- Avoiding silent failures
For structured approaches to error handling, check out this guide.
Writing effective unit tests
Clean code extends to unit tests. Effective tests:
- Follow the Arrange-Act-Assert (AAA) pattern.
- Focus on one behavior at a time.
- Have descriptive names to explain intent.
- Are easy to read and maintain.
More insights into writing meaningful tests can be found here.
Classes: single responsibility and cohesion
Well-designed classes:
- Follow SOLID principles.
- Maintain high cohesion and low coupling.
- Encapsulate behavior, exposing only necessary details.
- Adhere to dependency inversion, relying on abstractions rather than concrete implementations.
Concurrency: managing multiple threads safely
Concurrency introduces complexity, but well-structured concurrent code minimizes risks:
- Avoid shared mutable state.
- Use synchronization mechanisms like locks or atomic operations.
- Prioritize thread safety to prevent race conditions.
- Handle errors gracefully to maintain system stability.
Clean design principles: the foundation of maintainability
Beyond individual best practices, clean design involves following structured principles like:
- SOLID principles for modular and extensible code.
- Law of Demeter to limit unnecessary dependencies.
- Favoring composition over inheritance.
Final thoughts
Writing clean code is a continuous process, not a one-time task. Whether refining your code review approach, improving naming conventions, or optimizing error handling, each small step contributes to more maintainable and scalable software.
Let’s continue building software that’s clear, efficient, and enjoyable to work with. Happy coding!